Implicit Type Conversion Example in Java
Write a program to perform implicit type conversion
Try your Solution
Strongly recommended to Solve it on your own, Don't directly go to the solution given below.
Program or Solution
class Program
{
public static void main(String args[])
{
byte b = 10;
char ch = 'C';
short num = 500;
int i;
i = b;
System.out.println(i);
i = ch;
System.out.println(i);
i = num;
System.out.println(i);
num = b;
System.out.println(num);
//Following Conversions are not possible implictly because size of i is 4 bytes, ch is 2 bytes, b is 1 byte and num is 2 bytes
//b=i; // size of i is greater than b
//ch =i; // size of i is greater than ch
//b = num; // size of num is greater than b
//num = i; // size of i is greater than num
}
}
Output
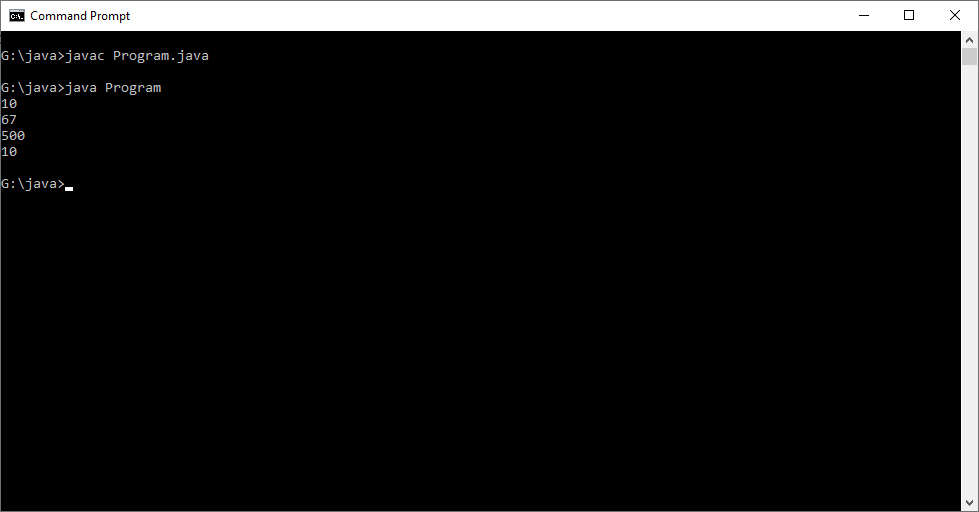
Program Explanation
Implicit Type casting take place when, the two types are compatible, the target type is larger than the source type.
The Commented lines are not possible because source types are larger than destination types.
Comments
Related Programs
- Java Program to Print Welcome
- Java Program to Print the given Message
- Java Program to Print the given word
- Java Program to print the given integer number
- Java Program to print the given fractional number
- Java Program to print the given fractional number in 2 digit decimal format
- Java Program to print the ASCII value of a character
- Java Program to print two numbers with a space between them
- Java Program to print two numbers with a tab space between them
- Java Program to print two numbers in two lines
- Java Program to Print the Character of given ASCII Value
- println Statement Example in Java
- Literals in Java With Example
- Print and println difference with Example
- Command Line Argument Example in Java
- Explicit Type Conversion Example in Java
- Escape Sequences and Format Specifiers Example in java