Java Program to Reverse the Elements of Array
Get array size n and n elements of array, then reverse the n elements.
Sample Input 1:
5
5 7 9 3 1
Sample Output 1:
1 3 9 7 5
Flow Chart Design
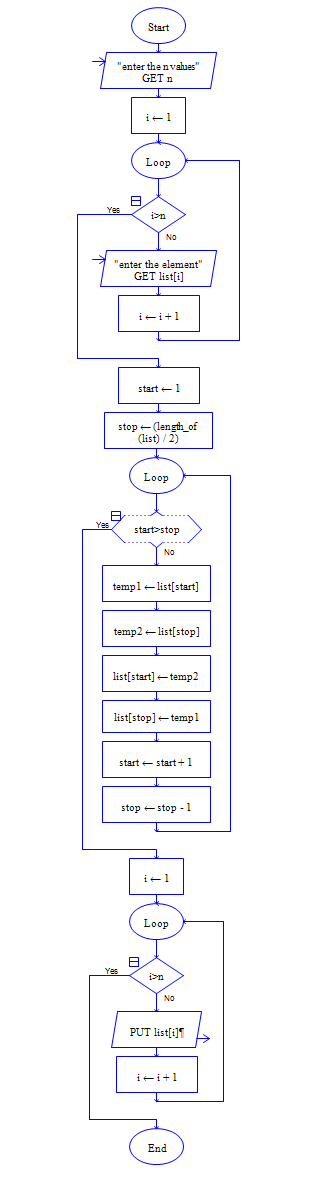
Try your Solution
Strongly recommended to Solve it on your own, Don't directly go to the solution given below.
public class Hello
{
public static void main(String args[])
{
//Write your code here
}
}
Program or Solution
//To Reverse The Elements In Array...
import java.util.*;
class Program
{
public static void main(String args[])
{
int size,i,j;
Scanner sc=new Scanner(System.in);
System.out.println("Enter The Size Of The Array:");
size=sc.nextInt();
int a[]=new int[size];
System.out.println("Enter The Array Elements:");
for(i=0;i<size;i++)
{
a[i]=sc.nextInt();
}
for(i=0,j=size-1;i<j;i++,j--)
{
int temp=a[i];
a[i]=a[j];
a[j]=temp;
}
System.out.println("The Output Is:");
for(i=0;i<size;i++)
{
System.out.println(" "+a[i]);
}
}
}
Program Explanation
Comments
Related Programs
- Java Program to get and print the array elements
- Java Program to find the sum of array elements.
- Java Program to find the average of array Elements
- Java Program to Search an Element in an array
- Java Program to find Largest element in the array
- Java Program to find Smallest element in the array
- Java Program to print all the numbers which are less than given key element from a given array.
- Java Program to Sort the Elements in ascending order.
- Java Program to Sort the Elements in descending order.
- Addition of Matrix in Java
- Java Program to delete an Element from the Array
- Java Program to merge two Arrays
- Java Program to Multiply two Matrices
- Java Program to Reverse the First Half Elements of Array
- Java Program to Reverse the Second Half Elements of Array
- Java Program to find the Second Largest Number in the Array
- Java Program to find the Second Smallest Number in the Array
- Java Program to transpose the Matrix
coming Soon
coming Soon