C Program to find the sum and average of array Elements
Get array size n and n elements of array, then compute sum and average of the elements.
Sample Input 1:
5 5 7 9 3 1
Sample Output 1:
25 5.0
Flow Chart Design
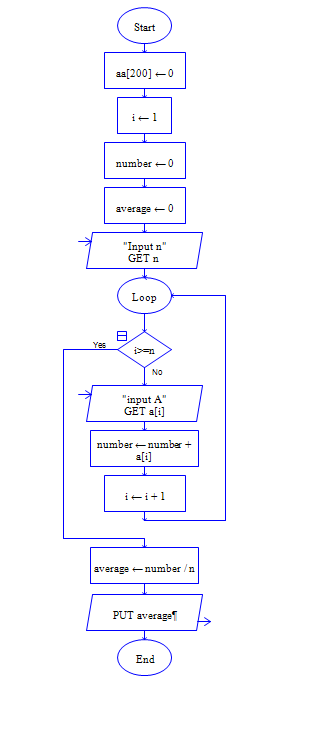
Try your Solution
Strongly recommended to Solve it on your own, Don't directly go to the solution given below.
#include<stdio.h>
int main()
{
//write your code here
}
Program or Solution
#include <stdio.h>
#include <stdlib.h>
int main(void)
{
int *a,n,i,sum=0,avg;
printf("Enter size of array:");
scanf("%d",&n);
a=malloc(sizeof(int)*n);
printf("Enter %d Elements:",n);
for(i=0;i<n;i++)
{
scanf("%d",&a[i]);
}
for(i=0;i<n;i++)
{
sum=sum+a[i];
}
avg=sum/n;
printf("\nSum of array:%d \nAverage of Array:%d",sum,avg);
return 0;
}
Program Explanation
i is initialized to 0 and incremented by 1 at each iteration of both the for loops.
First for loop reads n input numbers from user and stores them in array a[] from location 0 to n-1
Second for loop adds all the n elements of array located from 0 to n-1
Finally compute average using avg=sum/n statement and prints average avg using printf statement.
Comments
Related Programs
- C Program to get and print the array elements
- C Program to find the sum of array elements
- C Program to Search an Element in an array
- C Program to find Largest element in the array
- C Program to find Smallest element in the array
- C Program to print all the numbers which are less than given key element from a given array.
- C Program to find Second Largest element in the array
- C Program to find Second smallest element in the array
- C Program to delete an element in an array
- C Program to Reverse the Elements in array
- C Program to reverse the first half of array elements
- C Program to reverse the second half of array elements
- C Program to Sort the Elements in ascending order
- C Program to Sort the Elements in descending order
- C Program to sort half of the Array elements in ascending order and next half in descending order
- C Program to Merging two arrays
- C Program to replace every element with the greatest element on right side
- C Program to perform circular Array Rotation
- C Program to add two matrix
- C Program to multiply two matrix
- C Program to transpose the Matrix
coming Soon
coming Soon