Logical Operators Example in Java
Write a Program to illustrate Logical Operators in Java. The program should clearly demonstrate the working and supported data types of Logical Operator in Java.
These operators conduct logical "AND," "OR," and "NOT" operations, which are comparable to the AND and OR gates in digital electronics. They're used to integrate two or more conditions/constraints or to supplement the evaluation of a specific condition. One point to bear in mind is that if the first condition is false, the second condition is not examined, resulting in a short-circuiting effect. Used to test for a variety of conditions before deciding.
Try your Solution
Strongly recommended to Solve it on your own, Don't directly go to the solution given below.
Program or Solution
import java.util.Scanner;
class L_Operator
{
public static void main(String args[])
{
int num_1 = 10;
int num_2 = 20;
char ch_1 = 'D';
char ch_2 = 'E';
String name_1 = "Decode";
String name_2 = "Decode";
boolean val_1 = true;
boolean val_2 = false;
//Logical Operation on Numbers like int and float are not acceptable
//System.out.println(num_1 && num_2);
//System.out.println(num_1 || num_2);
//Logical Operation on Characters are not acceptable
//System.out.println(ch_1 && ch_2);
//System.out.println(ch_1 || ch_2);
//Logical Operation on Strings are not acceptable
//System.out.println(name_1 && name_2);
//System.out.println(name_1 || name_2);
//Logical Operation on boolean are allowed
System.out.println(val_1 && val_2);
System.out.println(val_1 || val_2);
System.out.println(!val_2); // unary Operator
//Logical operation on Relational Experession
System.out.println(num_1 < num_2 && num_1 != 0);
System.out.println(num_1 < num_2 || num_1 != 0);
System.out.println(!(num_1 < num_2)); // unary Operator
}
}
Output
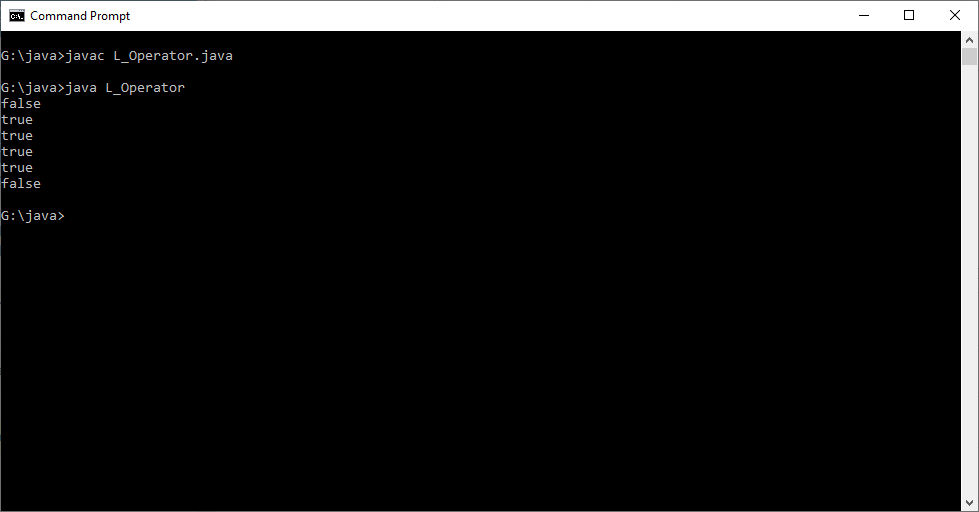
Program Explanation
Operator | Boolean | Expression Which Returns Boolean | Any Other Data Type |
Logical AND (&&) | ✓ | ✓ | |
Logical OR (||) | ✓ | ✓ | |
Logical Not (!) | ✓ | ✓ |
The Following Table Demonstrates the Data Type supported for Logical Operators.
Comments
Related Programs
- Java Program to Addition of two numbers
- Java Program to subtraction of two numbers
- Java Program to multiply two numbers
- Java Program to divide two numbers
- Java Program to find modulus of two numbers
- Java Program to Kilo Meters to Meters
- Java Program to Meters to Kilo Meters
- Java Program to find area of Square
- Java Program to find area of Rectangle
- Java Program to find area of Right-angled triangle
- Java Program to find area of triangle
- Java Program to find area of Circle (Use Constant)
- Java Program to find the distance between two points in 2D space
- Java Program to calculate kilobytes to bytes
- Java Program to calculate bytes to kilobytes
- Java Program to find simple interest
- Java Program to calculate Fahrenheit to Celsius
- Java Program to calculate Celsius to Fahrenheit
- Java Program to Swap two numbers using third variable
- Java Program to Swap of two numbers without using third variable
- Java Program to print the last digit of given number N
- Java Program to Calculate Salary for Employees
- Post Increment Operator Example in Java
- Pre Increment Operator Example in Java
- Pre Increment and Post Increment Difference in java with Example
- Ternary Operator Example in Java
- Relational Operators Example in java